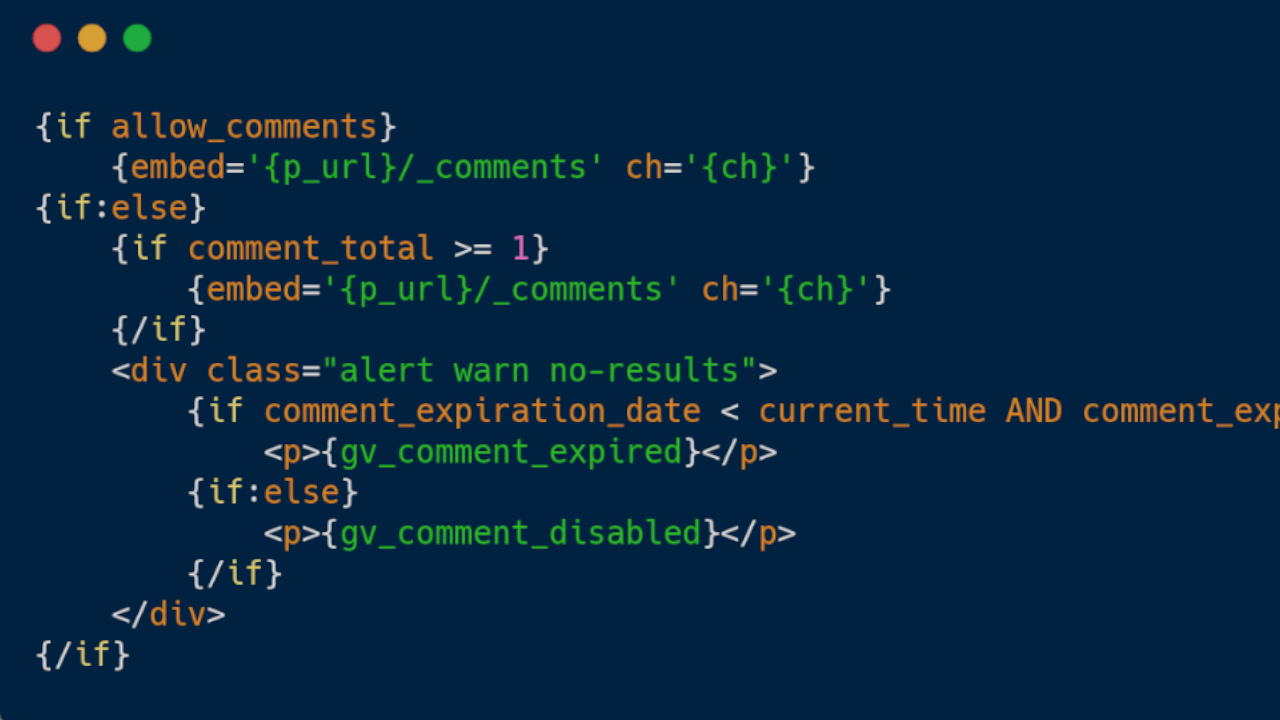
A Primer On Simple Conditionals
This article is intended as a gentle introduction to ExpressionEngine conditionals to give the reader an idea of how they work using real-world examples.
What is a “conditional”?
They allow you to compare or evaluate one piece of information against another and optionally show a response based on the result.
Conditionals generally work on the result being true or false - in essence, you’re asking a question to get an answer.
What can you compare/evaluate?
You can compare any variable or value available in ExpressionEngine, including global variables, channel entry fields, member fields, and your own custom values.
Here’s a non-exhaustive list of things you can evaluate either singly or one versus the other:
- numbers
- dates
- URL segments
- entry fields, title, custom fields
- files, filename, extension
- statuses
- trigger words (like yes/no, on/off)
- logged in status, name, email, etc.
- member group, name, ID, etc.
- your own values, hard coded or generated programmatically
When to use a conditional
There are near infinite uses, but some common ones are:
- checking if a visitor is logged in or not
- display alternative content based on the value of other content
- display different content based on part(s) of a URL
- changing HTML or other code based on the value of something
- adding opening and closing HTML tags like
<div></div>
- checking if something exists or not
- checking the value of something
Conditional tag syntax
Conditional tags are always wrapped in {if}{/if}
tags - just like regular HTML tags. You add values you want to evaluate and provide the answer based on the result.
Here are a few very simplistic examples showing you how evaluation logic works:
{if sky}Yes the sky exists!{/if}
{if sky is blue}It's day time{/if}
{if sky is black}It's night time{/if}
{if sky is NOT blue}It's night time{/if}
{if sky is NOT black}It's day time{/if}
See how we’re comparing the sky and its color then providing an answer for each variation? Note how there’s more than one way to provide the same answer (“is” and “is NOT”). The actual answer can be anything you want, even no answer at all.
Let’s start with a basic example
{if logged_in}
Hello customer
{/if}
What this does is check if the visitor has logged in to your site.
- if logged in, they will see the “Hello customer” message
- if not logged in, they will see nothing
Let’s expand that a bit more…
{if logged_in}
Hello customer
{if:else}
Please login
{/if}
What we’re now doing is getting two possible values.
- if logged in, they will see the “Hello customer” message
- if not logged in, they will see the “Please login” message
So “if” the first value is true do that, “else” do the other.
Evaluating entry content
Your entries are made up of several custom and core fields such as Title, Entry date, Status, and so on. You can evaluate any of these in a conditional, some are a little more complicated than others, but the logic is the same.
Let’s say you have a field for a blog excerpt contained in a div
element. Your template code may look like this:
<div class="row">
{blog_excerpt}
</div>
The only problem here; if the excerpt field is blank, the page will have an empty div
element in it; you won’t want that!
To solve this, you can use a conditional to only show the div
if the excerpt exists:
{if blog_excerpt}
<div class="row">
{blog_excerpt}
</div>
{/if}
So “if” the excerpt exists, then show it, otherwise do nothing. In other words, the
div
elements will only show if the excerpt exists.
Let’s expand that by providing a fallback message:
{if blog_excerpt}
<div class="row">
{blog_excerpt}
</div>
{if:else}
The author hasn't written an excerpt
{/if}
So “if” the excerpt exists, show it, “else” show the message.
Comparing a date
Date variables in ExpressionEngine allow you to format a display date however you need.
In this example, I’m using the 3 letter code for day of the week, Mon, Tue, Wed, etc. referenced by the %D
format value. The current_time
tag outputs, you can probably guess, the current time, as in right now.
The idea here is to show a message on a specific day, in this case, a Sunday:
{if "{current_time format='%D'}" == "Sun"}
Sorry we're closed today
{/if}
What this does is compare the current day of the week {current_time format="%D"}
to a target day value of “Sun”(day). If the answer is true (if today is Sunday) show the message, otherwise do nothing. The ==
value is shorthand for “is equal to.”
Note that here we have to wrap the current_time
tag in double quotes because it’s a tag inside another tag.
Testing against a URL segment
Conditionally do something based on part of a URL
Say you have a URL like https://example.com/blog/post/my-blog-post, then “blog” is segment 1, “post” is segment 2, and “my-blog-post” is segment 3 (See the docs for more on URL Segments ).
Here’s a couple of examples:
{if segment_1 == "blog"}This is the blog.{/if}
{if segment_2 == "post"}You're reading a blog post.{/if}
{if segment_1 == "about-us"}Why not read our blog?{/if}
{if segment_1 == ""}This must the home page.{/if}
Using a conditional to show a confirmation message
Say you have a contact form page at https://example.com/contact and your success page is at https://example.com/contact/thanks - you can run a conditional on segment_2 (thanks) to show a success message in the same template:
{if segment_2 == "thanks"}
Thanks for getting in touch!
{/if}
<form>
...contact form fields
</form>
Using a conditional to insert a CSS class name
Let’s say you wanted to add a class to an element but only in the /blog section. You could do something like this:
<body{if segment_1 == "blog"} class="blog"{/if}>
Would output as this (if the segment matched):
<body class="blog">
You could also append a new class name to an existing one like this:
<body class="body{if segment_1 == 'blog'} blog{/if}">
The result:
<body class="body blog">
Counting first and last
You can use conditionals to do simple calculations based on numbers. ExpressionEngine entries make available variables for:
count
(a running count of the entries being displayed, so the first entry will be “1”, the third entry would be “3”)total_results
(the total number of entries being displayed)
You want to mark the first or second item:
{if count == 1}
This is the first one
{/if}
{if count == 2}
This is the second one
{/if}
You want to mark the last item:
{if count == total_results}
This is the last one
{/if}
You can combine these when you need to output some HTML before and after your content:
{if count == 1}
<div class="row">
{/if}
Content...
{if count == total_results}
</div>
{/if}
Using operators
These allow you to compare things but in different ways. You can test for things that exist or don’t exist, less than or more than, and even look for specific words or phrases.
So far I’ve only used the ==
operator (equal to) in my examples. Here are a few more ways to compare values:
Check if something is not empty/blank
{if blog_exerpt != ""}The excerpt is not empty{/if}
Where != “” is asking if the field is not empty - not equals blank.
Check if something is empty/blank
{if blog_exerpt == ""}The excerpt is empty{/if}
Where == “” is asking if the field is empty - equals blank.
Check for a word or phrase in text
{if blog_exerpt *= "Tom"}The excerpt mentions Tom{/if}
Where *= is asking if the text contains the word “Tom.”
Check if something is less than x
{if product_stock < 10}Low stock{/if}
Where < is asking if the value is less than 10.
Check if something is more than x
{if product_stock > 10}We have loads in stock{/if}
Where > is asking if the value is more than 10.
There are many operators available for you to use. A full list is available in the documentation.
Troubleshooting conditionals
If your conditional tag doesn’t work, here are a few things to check:
- is the syntax correct?
- have you missed the trailing
{/if}
? - check spelling, missing or extra spaces
- is your tag in the right place in the template?
If you still have problems, it’s always worth posting on the EECMS Slack channel or the ExpressionEengine forums. An experienced eye will usually help you solve it.
Summing up
Conditionals can be hard to get your head around at first but stick with it. As soon as it clicks, you’ll find they can be incredibly powerful tools to help you create dynamic site content.
Comments 4
Gary Braid
Thanks Rob, that is an incredibly helpful article, just about to use the > operator, will save me quite a bit of time updating a free shipping message for products over a certain price on a clients site.
Any more brilliant vids on ee:u very welcome,
cheers Gary
Rob Allen
Glad you found it useful Gary!
jeffmace
Very helpful, I am just getting back into EE again, and this is helping to get me up to speed with the syntax. Thank you
anoopbal
Wow.. This article is so well-written and so understandable!! Please do write more Rob!