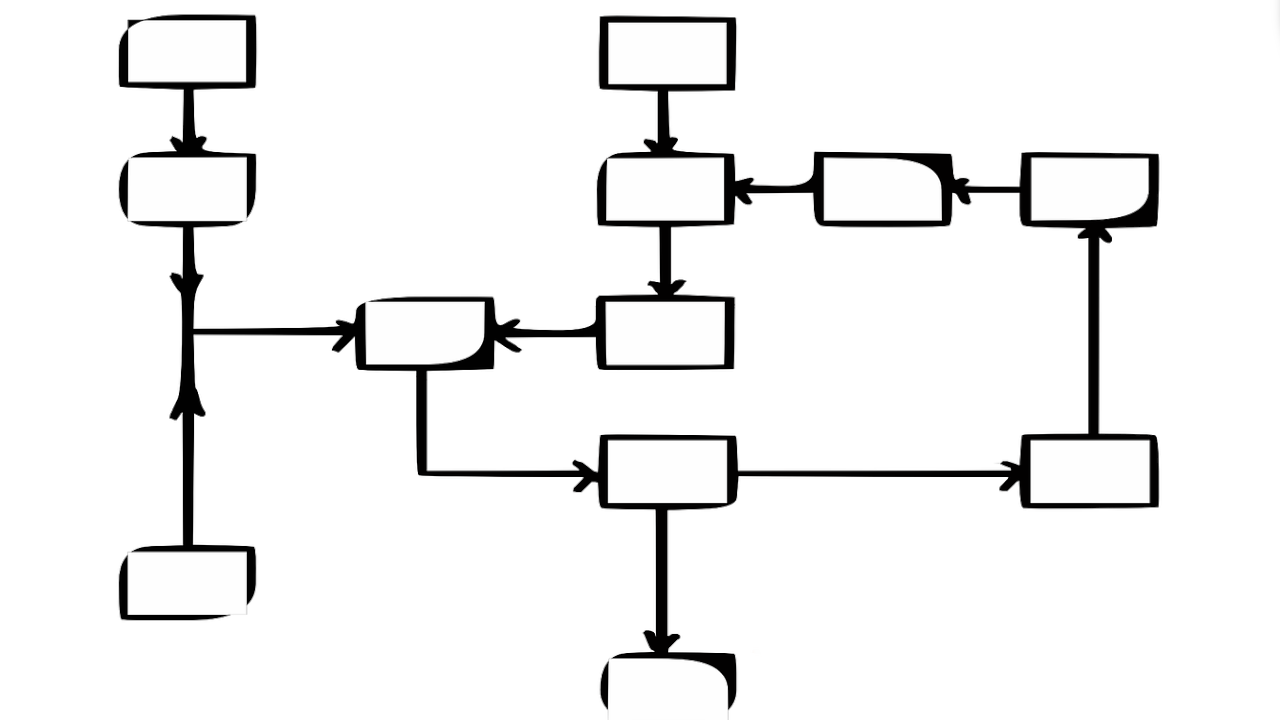
Advanced Conditionals Primer and Commonly Used Examples
This article assumes you understand how “simple” conditionals work.
Generally speaking a “simple” conditional lets you evaluate only two things in one way, “advanced” conditionals lets you evaluate many things in many ways.
There are near limitless ways to use advanced conditionals so instead of trying to cover everything, this article deals with commonly used examples and patterns.
Yes/no or true/false conditionals
Let’s say you have a custom field with values of “yes” and “no” and you want to display a custom message depending on the value, you can do something like this:
{if my_option_field == "yes"}
The answer is yes
{if:else}
The answer is no
{/if}
If the answer is yes show the yes message, else show the no message.
By using {if:else}
you tell ExpressionEngine to evaluate the first condition, if it’s true do something, if it’s not true do something else.
You could reverse what you evaluate first:
{if my_option_field == "no"}
The answer is no
{if:else}
The answer is yes
{/if}
If the answer is no show the no message, else show the yes message.
You could express this particular example as two simple conditionals:
{if my_option_field == "yes"}The answer is yes{/if}
{if my_option_field == "no"}The answer is no{/if}
For binary yes/no or true/false type answers either method will work fine.
What if you need a “don’t know” answer as well? You can evaluate two things with a fallback answer:
{if my_option_field == "yes"}
The answer is yes
{if:elseif my_option_field == "no"}
The answer is no
{if:else}
Don't know
{/if}
If the answer is yes show the yes message, else if the answer is no show the no message, else show the “don’t know” message.
You don’t always need the last {if:else}
, if you just wanted to output something for either value you could something like this:
{if my_option_field == "yes"}
The answer is yes
{if:elseif my_option_field == "no"}
The answer is no
{/if}
If the answer is yes show the yes message, else if the answer is no show the no message, otherwise do nothing
You can use as many if:elseif
statements as you need:
{if my_color == "red"}
I like red
{if:elseif my_color == "blue"}
I like blue
{if:elseif my_color == "green"}
I like green
{if:elseif my_color == "black"}
I like black
{if:elseif my_color == "white"}
I like white
{if:else}
I'm undecided
{/if}
Evaluating multiple values
On occasions you may want to evaluate various combinations of information to refine the answer. Here’s an example:
{if my_option_field == "yes" AND my_color_field == "blue"}
Yes, my favourite color is blue
{/if}
If the answer is yes and blue output the message, otherwise do nothing.
The AND
logical operator lets you evaluate two parameters, so if both are true you get a result, if none or neither are true nothing happens.
You can evaluate as many parameters as you want:
{if my_option_field == "yes" AND my_color_field == "blue" AND my_date_field == "Friday"}
Yes, my favourite color is blue, but only on Friday
{/if}
If the answer is yes and the colour is blue but only show the result of the day is Friday.
You can use other comparisons instead of ==
(equals to) such as this one that uses !=
(not equal to):
{if my_option_field == "yes" AND my_color_field != "blue"}
Yes, but my favourite color is not blue
{/if}
If the answer is yes and the colour is not blue output the message, otherwise do nothing.
You can always include custom field tags to output values dynamically:
{if my_option_field == "yes" AND my_color_field == "blue" my_date_field == "Friday"}
{my_option_field}, my favourite colour is {my_color_field}, but only on a {my_date_field}
{/if}
Conditionals with dates
In these examples I’m using the {current_time}
variable to get the date values in tandem with the various date format values that are available. Note how the {current_time}
variable is wrapped in double quotes, and the format parameter value is wrapped in single quotes. While this is often the case, conditionals can sometimes work without them. If unsure test both ways!
Compare the current year
{if "{current_time format='%Y'}" == "2021"}
It's 2021
{if:else}
It's not 2021
{/if}
Compare a specific date
You can be more specific with the date format, in this example it will only output the message if the current date is 1st January.
{if "{current_time format='%d/%m'}" == "01/01"}
It's the first day of the year
{/if}
Compare the current day name
{if "{current_time format='%D'}" == "Sun"}
Sorry we're closed on Sundays
{/if}
Compare current, past and future
In this example I’m using the <
(less than) and >
(greater than) logical operators.
{if "{current_time format='%Y'}" == "2021"}
It's 2021
{if:elseif "{current_time format='%Y'}" < "2021"}
It's so last year
{if:elseif "{current_time format='%Y'}" > "2021"}
This is the future if you hit 88mph
{/if}
Comparing an entry date
In this example I’m evaluating whether an entry was published this year or in a previous years.
{if "{current_time format='%Y'}" == "{entry_date format='%Y'}"}
Published this year
{if:elseif "{current_time format='%Y'}" < "{entry_date format='%Y'}"}
This entry is old hat
{/if}
Nesting conditionals
You can evaluate one conditional inside another:
{if my_option_field == "yes"}
My favourite color is:
{if my_color_field == "red"}
red
{if:elseif my_color_field == "blue"}
blue
{if:elseif my_color_field == "green"}
green
{if:else}
undecided
{/if}
{if:elseif my_option_field == "no"}
not specified
{/if}
Bear in mind that nesting conditionals many layers deep can sometimes have an impact on performance - the deeper you nest the more database queries may be needed or EE needs more memory to parse the template contents. As usual with these things it depends on what you’re doing, but if you add some deeply nested conditionals and get slower loading pages it could be a culprit.
Commonly used conditions in a channel entries tag
Statuses
On occasions you may want to evaluate combinations of information to refine the output. Here’s an example:
{if status == "featured"}
Featured article
{if:elseif status == "open"}
This article is merely open, not featured
{/if}
Different content for logged in members
Lets start by just checking if someone from any member role is logged in or not:
{if logged_in}
Member content
{if:else}
This page is private, please login
{/if}
Or if you need the messages in two different places you could do simple conditionals:
{if logged_in}
Member content
{/if}
{if logged_out}
This page is private, please login
{/if}
Restricting content by member role (group)
{if logged_in_role_id == "7"}
Gold member!
{if:elseif logged_in_role_id == "8"}
Silver member
{if:elseif logged_in_role_id == "9"}
Bronze member
{if:elseif logged_in_role_id == "1"}
Super admin!
{/if}
Summing up
Hopefully these examples will help solve some of your advanced conditional problems, or at least point you in the right direction.
Comments 2
vw000
Great article Rob!
hapax63615
There are countless ways to use advanced conditionals that you have helped me understand Quick Draw better.