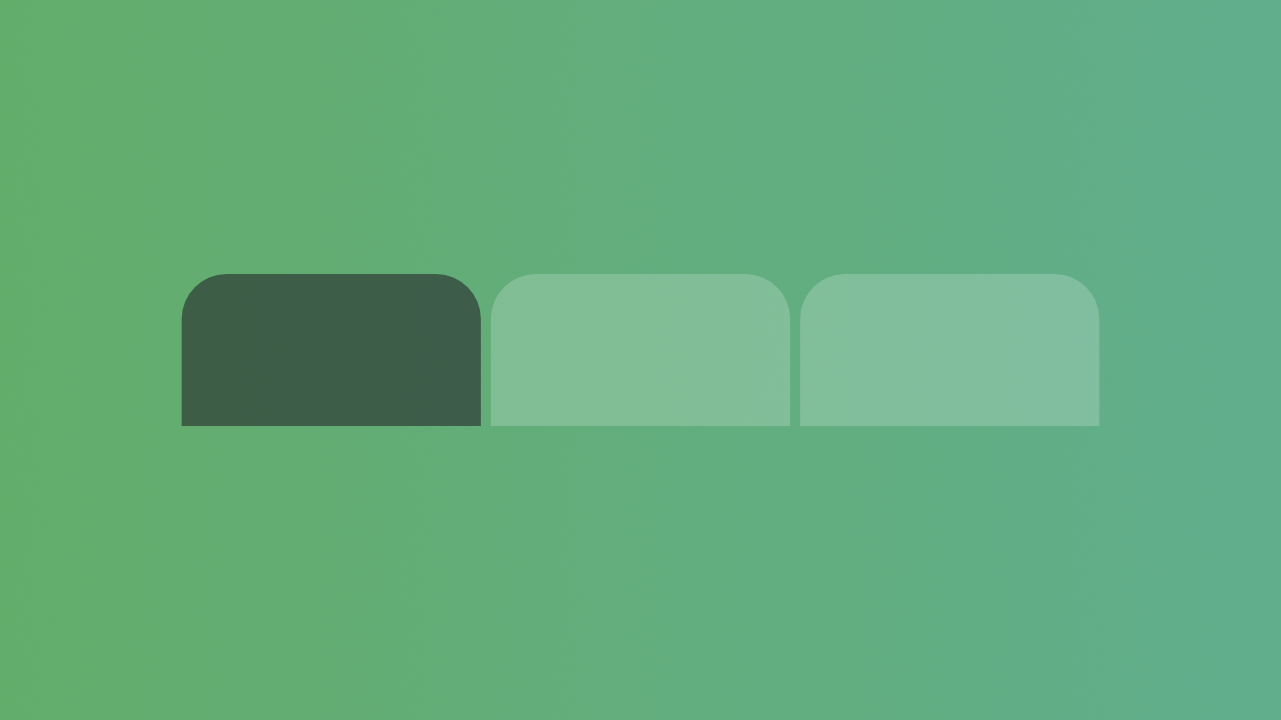
Content Tabs
First we need some HTML to tab!
<div class="tabs-wrap">
<ul class="tabs">
<li><a class="act" href="" rel="t-0">Tab 1</a></li>
<li><a href="" rel="t-1">Tab 2</a></li>
<li><a href="" rel="t-2">Tab 3</a></li>
</ul>
<div class="tab-content t-0 tab-open">
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.</p>
</div>
<div class="tab-content t-1">
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.</p>
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.</p>
</div>
<div class="tab-content t-2">
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</p>
</div>
</div>
We use an array indexing numbering scheme, so the first tab is t-0
, then t-1
and so on. tab-open
indicates the default, open tab. You can move this to any tab-content
, but only one tab-content
may be chosen at a time. As you can see in the tabs each is assigned a rel=
that matches the tab-content
it should open and close.
Now we’re going to need some light styles to give this a tabbed look. First we need a tab CSS object.
.tabs{
list-style-type: none;
margin: 0;
overflow: hidden;
padding: 0;
li{
float: left;
}
}
.tab-content{
display: none;
p, ul, ol{
&:last-child{
margin-bottom: 0;
}
}
}
.tab-open{
display: block;
}
And for visuals the tab component CSS.
.tabs{
margin-top: 20px;
a{
background-color: #F9F9F9;
display: inline-block;
line-height: 1;
padding: 10px;
&.act,
&:hover{
background-color: #EEEEEE;
}
}
}
.tab-content{
background-color: #EEEEEE;
margin-bottom: 20px;
padding: 1px 20px 20px;
.tab-content{
background-color: #FFFFFF;
margin-bottom: 0;
}
.tabs{
a{
&.act,
&:hover{
background-color: #FFFFFF;
}
}
}
}
And last but most !important
we’ll need some javascript to make the tabs function as expected.
$('.tabs-wrap > .tabs a').on('click',function(){
var tabClassIs = $(this).attr('rel');
$('.tb-act').removeClass('tb-act');
$(this)
.closest('ul')
.closest('.tabs-wrap')
.addClass('tb-act');
// close OTHER .tab(s), ignores the currently open tab
$('.tb-act > .tabs a')
.not(this)
.removeClass('act');
// removes the .tab-open class from any open tabs, and hides them
$('.tb-act > .tab-content')
.not('.tab-content.'+tabClassIs+'.tab-open')
.removeClass('tab-open');
$(this).addClass('act');
$('.tb-act > .tab-content.'+tabClassIs).addClass('tab-open');
// stop THIS from reloading
// the source window and appending to the URI
// and stop propagation up to document
return false;
});
You can demo and play with this code here: https://jsfiddle.net/jmathias/spktuvff/
You can also nest tab-wrap
inside a tab-content
and have multiple tab-wraps
per HTML document.
That’s that, a quick and simple way to add tabbed content to your next ExpressionEngine site!
Comments 0
Be the first to comment!