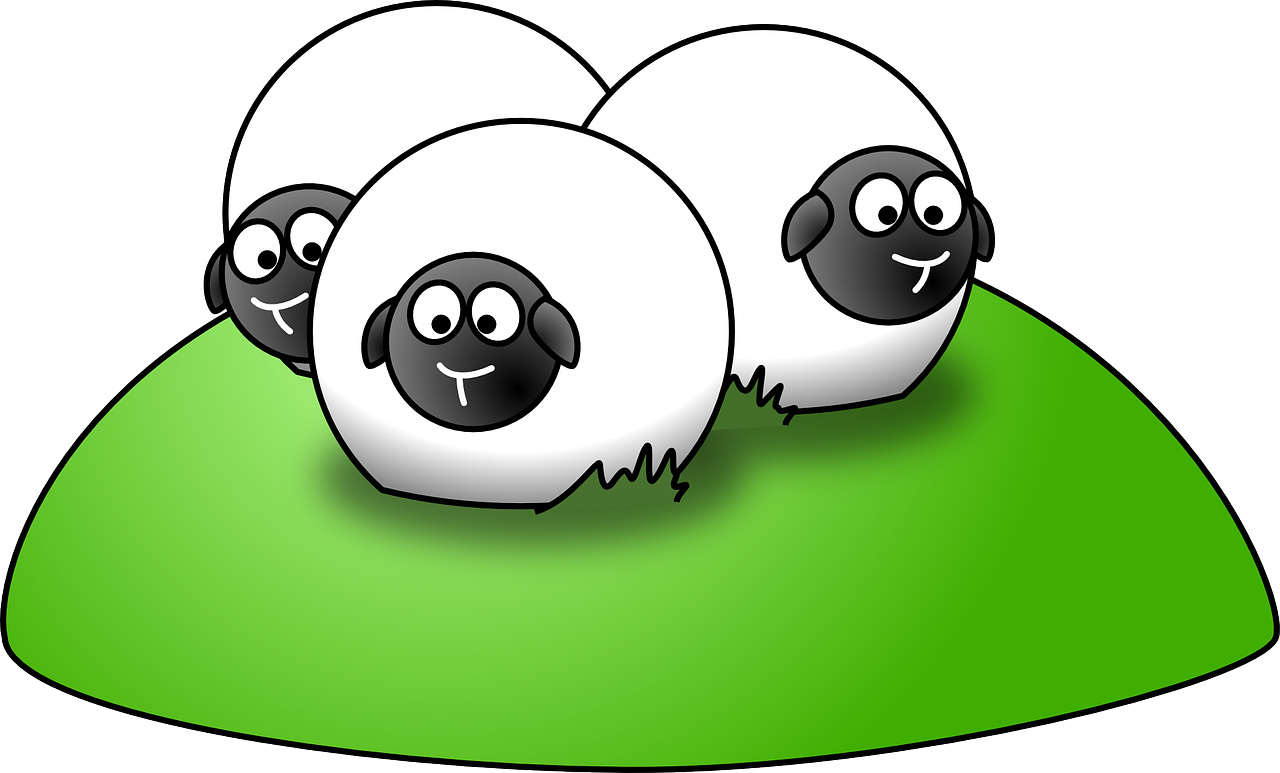
Ultra Double Secret Manual: Shared Form Part Two
All About All The Fields
Here they are. Every single field option with every available setting. What you can do and what you get from it. Minimal snark.
Note that this is Part 2 of a series on the ExpressionEngine Shared Form View libraries. It’s assumed you’ve read Part One so if you haven’t yet, go nuts.
If it gets overwhelming, just remind yourself, “not all variables are needed depending on the ‘type’ value”. It’ll help. Maybe. What do I know.
Also, keep your pets nearby for moral support.
Universal Variable
Oh. Right. Yeah. There are 2 of them :shrug-emoji:
Variable | Definition | Type | Required |
---|---|---|---|
name |
The value to use for the name parameter of the input field |
string | No |
type |
One of a ton of varying values, described below | string | Yes |
Most Variables
These will work in about 90% of situations. FWIW, the parser doesn’t really care if you have variables for Fields that aren’t used. So there’s no real worry of anything breaking if you, for example, use the no_results
variable on the text
input field.
Variable | Definition | Type | Required |
---|---|---|---|
class |
Any specific CSS class to apply. | string | No |
margin_top |
Adds the CSS class add-mrg-top to the containing div |
boolean | No |
margin_left |
Adds the CSS class add-mrg-left to the containing div |
boolean | No |
value |
The data to populate the Field | string | No |
required |
Denotes the field visually as being required for validation | boolean | No |
attrs |
A complete key="value" string to apply to the field |
string | No |
disabled |
Whether the field can accept input/editing | string | No |
group_toggle |
Used in conjunction with group to hide/show field sets. See the docs for details |
string | No |
maxlength |
Hard lock a field to only allow XX characters | integer | No |
placeholder |
The string to use for the input fields placeholder parameter |
string | No |
group |
Used in conjunction with group_toggle to hide/show field sets. See the docs for details |
string | No |
note |
A raw string of text to output BENEATH the field | string | No |
no_results |
Used to define quick links when choices are empty |
array | No |
choices |
A simple key=>value array pair to populate options |
array | No |
no_results
The no_results
variable should follow the format of:
[
'text' => '',
'link_href' => '',
'link_text' => '',
]
A Complete List of Fields
And now we’re here. The whole freaking point. All the fields. Leggo.
text
short-text
file
password
hidden
textarea
action_button
html
select
toggle
dropdown
checkbox
radio
multiselect
slider
image
text
Adds a traditional input
HTML field.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'text'
],
]
Output
<input type="text" name="FIELD_NAME" value="">
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'text',
'class' => 'my-custom-class', //note this is applied to wrapping div on input
'attrs' => ' custom-param="12" ',
'value' => '[email protected]',
'maxlength' => 24,
'placeholder' => 'Your Email Here',
'note' => 'Another Place for copy',
'disabled' => false, //note if true the `attrs` attribute is ignored
],
]
Output
<input type="text" name="FIELD_NAME" value="[email protected]" custom-param="12" maxlength="24" placeholder="Your Email Here">
short-text
Adds a small input
HTML field wrapped in a div with flex-input
as the class. Useful for stacking fields horizontally.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'short-text'
],
]
Output
<label class="flex-input ">
<input type="text" name="FIELD_NAME" value="">
</label>
Variables
The short-text
field only has 1 custom variable lol
Variable | Definition | Type | Required |
---|---|---|---|
label |
Will place a bold string under the field for users to clickety clackity on | string | No |
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'short-text',
'class' => 'my-custom-class', //note this is applied to wrapping label on input
'attrs' => ' custom-param="12" ',
'value' => '[email protected]',
'maxlength' => 24,
'placeholder' => 'Your Email Here',
'label' => 'FIELD_LABEL_VALUE',
'note' => 'Another Place for copy',
'disabled' => false, //note if true the `attrs` attribute is ignored
],
]
Output
<label class="flex-input my-custom-class">
<input type="text" name="FIELD_NAME" value="[email protected]" custom-param="12" maxlength="24" placeholder="Your Email Here">
<span class="label-txt">FIELD_LABEL_VALUE</span>
</label>
file
Adds a traditional file
HTML field to your form.
Note that to use the
file
field you’ll have to set'has_file_input' => true
in the view level variables you pass toee:_shared/form
so the formenctype
is changed to allow file uploads.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'file',
],
],
Output
<input type="file" name="FIELD_NAME" class="">
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'file',
'class' => 'my-custom-class', //note this is applied to the input element
'attrs' => ' custom-param="12" ',
'note' => 'Another Place for copy',
'disabled' => false, //note if true the `attrs` attribute is ignored
],
]
Output
<input type="file" name="FIELD_NAME" disabled="disabled" class="my-custom-class">
password
Adds your run of the mill password
input field wrapped in magic that puts an eye in it users can click to deobfuscate the input.
It’s important to note the value you use for the
name
variable. If the value isverify_password
orpassword_confirm
, theautocomplete
parameter iscurrent-password
otherwise the value isnew-password
.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'password'
],
],
Output
<input type="password" name="FIELD_NAME" value="" autocomplete="new-password" class="">
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'password',
'class' => 'my-custom-class', //note this is applied to the input field
'attrs' => ' custom-param="12" ',
'value' => 'THE_VALUE',
'maxlength' => 24,
'placeholder' => 'PLACEHOLDER_VALUE',
'note' => 'NOTE_STRING',
'disabled' => false, //note if true the `attrs` attribute is ignored
],
]
Output
<input type="password" name="FIELD_NAME" value="THE_VALUE" autocomplete="new-password" custom-param="12" maxlength="24" placeholder="PLACEHOLDER_VALUE" class="my-custom-class">
hidden
Adds a hidden field to your form.
Note that if you care about visual dissonance, you REALLY don’t want to use this stand-alone in your form. It throws the spacing off. Like, WAY off. It’ll legit just look weird. Either append it to an existing field group at the bottom of your form (last element, basically), OR define your hidden fields in your view variables.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'hidden',
'value' => 'VALUE'
],
]
Output
<input type="hidden" name="FIELD_NAME" value="VALUE">
All Options
There are ony 4 for, hopefully, obvious reasons.
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'hidden',
'value' => 'THE_VALUE',
'note' => 'NOTE_STRING',
],
]
Output
<input type="hidden" name="FIELD_NAME" value="THE_VALUE">
textarea
Adds a full textarea
input field.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'textarea'
],
]
Output
<textarea name="FIELD_NAME"></textarea>
Variable | Definition | Type | Required |
---|---|---|---|
cols |
How many columns to set the field to use | int | No |
rows |
The total rows | int | No |
kill_pipes |
Flag to replace any ¦ delimiters with new lines (\n ) |
boolean | No |
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'textarea',
'class' => 'my-custom-class', //note this is applied to input field
'attrs' => ' custom-param="12" ',
'value' => 'THE_VALUE',
'maxlength' => 24,
'placeholder' => 'PLACEHOLDER_VALUE',
'note' => 'NOTE_STRING',
'disabled' => false, //note if true the `attrs` attribute is ignored
'cols' => 20,
'rows' => 30,
'kill_pipes' => false,
],
]
Output
<textarea name="FIELD_NAME" cols="20" rows="30" custom-param="12" maxlength="24" placeholder="PLACEHOLDER_VALUE">THE_VALUE</textarea>
action_button
Adds a “pretty” button style link to your form.
Note that
action_button
requires 2 additional variables by default.
Variable | Definition | Type | Required |
---|---|---|---|
link |
The full URL you want to use | string | Yes |
text |
The copy to use for the button | string | Yes |
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'action_button',
'link' => 'https://u.expressionengine.com/',
'text' => 'Ultra Double Secret Manual for ExpressionEngine Shared Form View Part Two'
],
]
Output
<a class="button button--secondary tn " href="https://u.expressionengine.com/">Ultra Double Secret Manual for ExpressionEngine Shared Form View Part Two</a>
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'action_button',
'link' => 'https://u.expressionengine.com/',
'text' => 'Ultra Double Secret Manual for ExpressionEngine Shared Form View Part Two',
'class' => 'my-custom-class', //note this is applied to wrapping div on input
'note' => 'NOTE_STRING',
],
]
Output
<a class="button button--secondary tn my-custom-class" href="https://u.expressionengine.com/">Ultra Double Secret Manual for ExpressionEngine Shared Form View Part Two</a>
html
Allows for putting raw (unformatted) HTML inside your form.
Note that
html
requires 1 additional variable by default.
Variable | Definition | Type | Required |
---|---|---|---|
content |
The HTML ahem content you want to output | string | Yes |
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'html',
'content' => '<strong>my string</strong>'
],
]
Output
<strong>my string</strong>
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'html',
'content' => '<strong>my string</strong>',
'class' => 'my-custom-class', //note this is applied to wrapping div around the content
'note' => 'NOTE_STRING',
],
]
Output
<div class="my-custom-class">
<strong>my string</strong>
</div>
select
Adds a traditional select
input field to your form.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'select',
'choices' => [
1 => 'One',
2 => 'Two',
3 => 'Three'
]
],
]
Output
<select name="FIELD_NAME" class="">
<option value="1">One</option>
<option value="2">Two</option>
<option value="3">Three</option>
</select>
Variable | Definition | Type | Required |
---|---|---|---|
encode |
Whether to format text so that it can be safely placed in a form field in the event it has HTML tags. | boolean | No |
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'select',
'choices' => [
1 => 'One',
2 => 'Two',
3 => 'Three'
],
'class' => 'my-custom-class', //note this is applied to input field
'attrs' => ' custom-param="12" ',
'value' => 'THE_VALUE',
'note' => 'NOTE_STRING',
'disabled' => false, //note if true the `attrs` attribute is ignored
'no_results' => [
'text' => 'Nothing Here',
'link_href' => 'URL_TO_ADD_OPTION',
'link_text' => 'Add Option'
],
],
]
Output
<select name="FIELD_NAME" custom-param="12" class="my-custom-class">
<option value="1">One</option>
<option value="2">Two</option>
<option value="3">Three</option>
</select>
toggle
/ yes_no
Adds a Toggle control that returns either y or n respectively when yes_no
is used or a traditional boolean when toggle
is used.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'yes_no', // | toggle
],
]
Output
<button type="button" class="toggle-btn off yes_no " data-toggle-for="FIELD_NAME" data-state="off" role="switch" aria-checked="false" alt="off">
<input type="hidden" name="FIELD_NAME" value="n">
<span class="slider"></span>
</button>
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'yes_no', // | toggle
'class' => 'my-custom-class',
'value' => 'THE_VALUE',
'note' => 'NOTE_STRING',
'disabled' => false,
],
]
Output
<button type="button" class="toggle-btn off yes_no my-custom-class" data-toggle-for="FIELD_NAME" data-state="off" role="switch" aria-checked="false" alt="off">
<input type="hidden" name="FIELD_NAME" value="n">
<span class="slider"></span>
</button>
dropdown
Adds a fancy select input field especially useful for large data sets to choose from.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'dropdown',
'choices' => [
1 => 'One',
2 => 'Two',
3 => 'Three',
4=> 'Four',
]
],
]
All Options
Variable | Definition | Type | Required |
---|---|---|---|
limit |
Magic | integer | No |
filter_url |
Magic | string | No |
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'dropdown',
'value' => 2,
'limit' => 100,
'empty_text' => 'Whatever you want',
'filter_url' => '',
'choices' => [
1 => 'One',
2 => 'Two',
3 => 'Three',
4=> 'Four',
],
'no_results' => [
'text' => 'Nothing Here',
'link_href' => 'URL_TO_ADD_OPTION',
'link_text' => 'Add Option'
],
],
]
checkbox
Adds a specialized widget for checkbox
.
Note that if you have more than 8 options the widget becomes a unicorn with a beautiful horn.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'checkbox',
'choices' => [
1 => 'One',
2 => 'Two',
3 => 'Three',
],
],
]
All Options
Variable | Definition | Type | Required |
---|---|---|---|
disabled_choices |
A list of keys used in the options array to prevent selection |
array | No |
selectable |
Whether to allow user interaction with the field | boolean | No |
reorderable |
Allow drag and drop ordering of the options? | boolean | No |
removable |
Adds UI element to remove options | boolean | No |
encode |
Whether to format text so that it can be safely placed in a form field in the event it has HTML tags. | boolean | No |
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'checkbox',
'value' => [2,1],
'choices' => [
1 => 'One',
2 => 'Two',
3 => 'Three',
],
'disabled_choices' => [
3
],
'selectable' => true,
'reorderable' => false,
'removable' => false,
'editable' => false,
'encode' => true,
'attrs' => ' custom-param="12" ',
'no_results' => [
'text' => 'Nothing Here',
'link_href' => 'URL_TO_ADD_OPTION',
'link_text' => 'Add Option'
],
],
]
radio
This behaves just like checkbox
with the exception of being scalar so uses a scalar for the value. See checkbox
for details.
Note you can alias this with
radio_block
orinline_radio
for reasons
multiselect
Deploys a series of select
input fields together.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'multiselect',
'choices' => [
'bday_day' => [
'label' => 'Day',
'choices' => [],
'value' => ''
],
'bday_month' => [
'label' => 'Month',
'choices' => [],
'value' => ''
],
'bday_year' => [
'label' => 'Year',
'choices' => [],
'value' => ''
]
]
],
]
Output
<div class="field-inputs">
<label>
Day<select name="bday_day"></select>
</label>
<label>
Month<select name="bday_month"></select>
</label>
<label>
Year<select name="bday_year"></select>
</label>
</div>
All Options
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'multiselect',
'note' => 'MY_NOTE',
'choices' => [
'bday_day' => [
'label' => 'Day',
'choices' => [],
'value' => ''
],
'bday_month' => [
'label' => 'Month',
'choices' => [],
'value' => ''
],
'bday_year' => [
'label' => 'Year',
'choices' => [],
'value' => ''
]
]
],
]
Output
<div class="field-inputs">
<label>
Day<select name="bday_day"></select>
</label>
<label>
Month<select name="bday_month"></select>
</label>
<label>
Year<select name="bday_year"></select>
</label>
</div>
slider
Puts a slider widget into your form
Note that this field appears to still be expirmental. Hence why it’s not “officially” documented.
Basic Example
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'slider',
],
]
Output
<input type="range" rel="range-value"
id="FIELD_NAME"
name="FIELD_NAME"
value=""
min="0"
max="100"
step="1"
>
<div class="slider-output"><output class="range-value" for="FIELD_NAME"></output>%</div>
All Options
Variable | Definition | Type | Required |
---|---|---|---|
min |
The lowest value in the range of permitted values | integer | No |
max |
The greatest value in the range of permitted values | integer | No |
step |
Specifies the granularity that the value must adhere to (if any is used, no stepping is implied, and any value is allowed (barring other constraints, such as min and max ) |
mixed | No |
unit |
The symbol your data can best be represented by (defaults to % ) |
mixed | No |
'fields' => [
'FIELD_NAME' => [
'name' => 'FIELD_NAME',
'type' => 'slider',
'min' => 100,
'note' => 'MY_NOTE',
'max' => 400,
'step' => 3,
'unit' => '$',
],
]
Output
<input type="range" rel="range-value"
id="FIELD_NAME"
name="FIELD_NAME"
value=""
min="100"
max="400"
step="3"
>
<div class="slider-output"><output class="range-value" for="FIELD_NAME"></output>$</div>
image
It doesn’t exist. Don’t believe anyone. There is no image
field.
Instead, take a look at this Forum post about how to get a head start on a future Part.
I know, pretty unsatisfying, but, I mean, whatever :shakes-head:
Wrapping Up
At this point, you should know everything you need to know to create a form using the Shared/Form View libraries. There are a couple more points to cover, for example adding Ajax requests and Grid Fields and Tables, but these are the high notes.
Next steps is to focus on form processing, Model objects, and Validation. Which should be a lot more fun.
Comments 0
Be the first to comment!